Flutter 개념 용어 정리
- pubspec.yaml : Flutter를 운영하는데 필요한 라이브러리를 모아둔 파일이라고 보면 된다.
- StatelessWidget : 상태가 변화하지 않는 화면. 즉, Build메소드가 최초의 1회만 호출된다
-> 클래스는 1개로 이루어져있다. (class 클래스명 extends StatelessWidget) - StatefulWidget : 상태가 변화하는 화면. 즉, Build 메소드는 요청될때마다 호출된다.
-> 클래스는 2개로 이루어져있다. (class 클래스명 extends StatefulWidget 과 클래스명State extends State<클래스명>) - setState : StatefulWidget과 세트. StatefulWidget에서 Build 메소드를 호출할때 사용된다.
StatelessWidget과 StatefulWidget은 어떻게 운용이되나? 예를 들면 다음과 같다.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: HelloPage('Hello World'));
}
}
class HelloPage extends StatefulWidget {
final String title;
HelloPage(this.title);
@override
_HelloPageState createState() => _HelloPageState();
}
class _HelloPageState extends State<HelloPage> {
@override
Widget build(BuildContext context) {
return Scaffold(
floatingActionButton: FloatingActionButton(
child: Icon(Icons.add), onPressed: () => print('잘 눌렸다.')),
appBar: AppBar(title: Text(widget.title)),
body: Text(widget.title, style: TextStyle(fontSize: 30)));
}
}
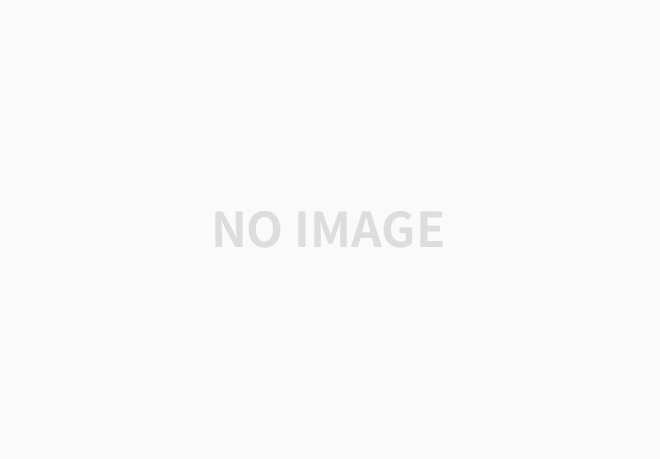
- private 변수 : 일반적으로 입력하면 public 변수가 되지만, 변수명에 '_'을 추가로 입력하면 private 변수가 되어 해당 변수가 선언된 클래스 내에서만 사용이 가능하다. (ex : string _message = 'Hello World!';)
class _HelloPageState extends State<HelloPage> {
String _message = 'Hello World!';
@override
Widget build(BuildContext context) {
return Scaffold(
floatingActionButton: FloatingActionButton(
child: Icon(Icons.add), onPressed: _changeMessage),
appBar: AppBar(title: Text(widget.title)),
body: Text(_message, style: TextStyle(fontSize: 30)));
}
void _changeMessage() {
setState(() {
_message = '안녕하세요?';
});
}
}
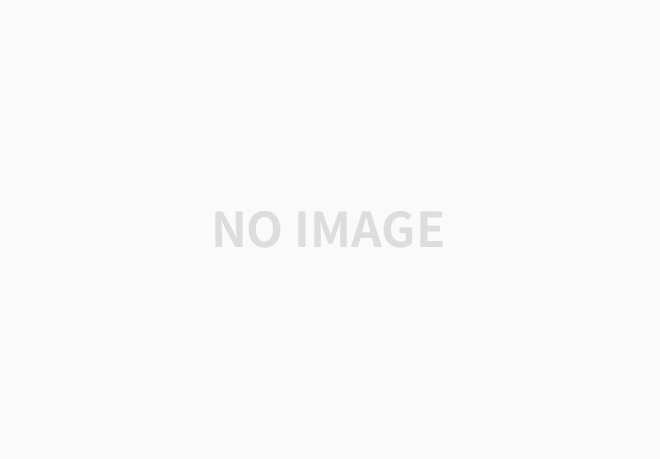
- int형을 text로 출력 : Text('$변수명')을 선언하면된다.
Text('$_counter', style: TextStyle(fontSize: 30)),
- Column과 Row 구조 : Column / Row안에 -> 본인이 원하는 정렬 상태 -> Children이 들어간다.
Column과 Row자체가 여러가지를 나열한단 의미이므로, Children이 들어가게 된다.
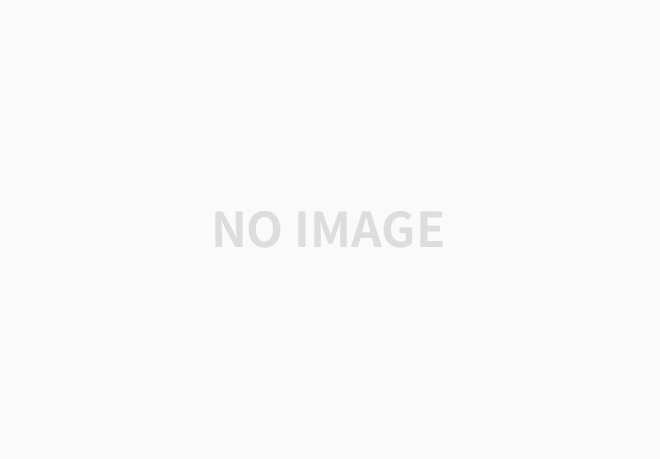
- Stack : 자식요소들이 겹쳐서 들어오게 된다.
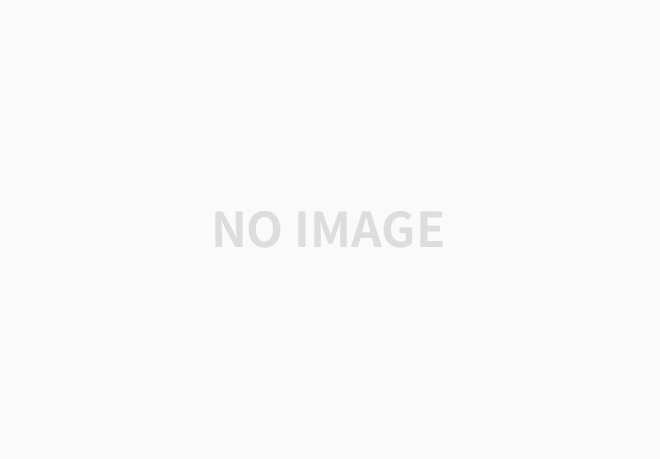
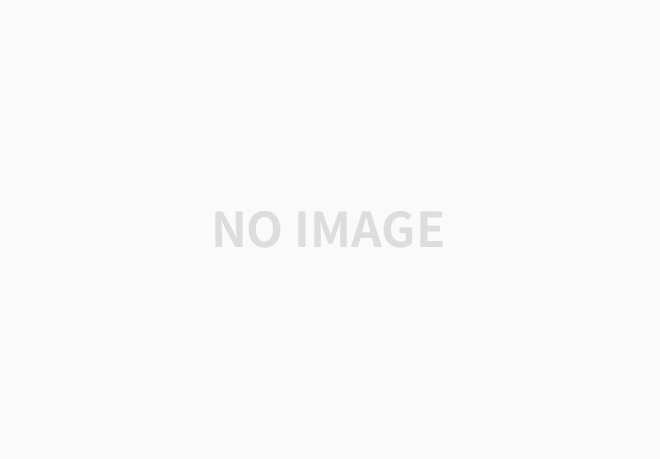
- 화면 전환 네비게이션 : 화면이 떠오르면서 새로운 화면으로 전환되는 경우, Navigator.push를 이용하여 화면을 전환한다.
반대로 화면이 빠지면서 새로운 화면으로 전환되는 경우, Navigator.pop을 사용한다.
... // 생략
children: <Widget>[
Text(_message, style: TextStyle(fontSize: 30)),
Text('$_counter', style: TextStyle(fontSize: 30)),
RaisedButton(
child: Text('화면이동'),
onPressed: () {
Navigator.push(context, //context의 경우, override과정에서 필요할 경우 값이 전달된다.
MaterialPageRoute(builder: (context) => CupertinoPage()));
},
)
],
)));
'📱 Mobile > Flutter' 카테고리의 다른 글
Flutter 기본 개념 - 인스타그램 클론 코딩 (0) | 2020.10.04 |
---|---|
Meterial 디자인 vs Cupertino 디자인 (0) | 2020.10.04 |
Flutter 로그인 창 화면 구현 (0) | 2020.09.24 |